- Error Zero
- Posts
- 5 Simple Ways to Debug Like a Pro for Every Coder Out There
5 Simple Ways to Debug Like a Pro for Every Coder Out There
Hey folks, let's face it, as much as we'd like our code to be flawless, errors are like that annoying friend who crashes the party uninvited. But don't worry, debugging is your superpower! Every dev should get good at it because, let's be real, it saves you a ton of time and makes you a coding ninja. So, here are five easy-peasy debugging tricks to get you started:
1. Get to Know the Error Like it's Your BFF
First things first, take a chill pill and get to know the error. It's like solving a puzzle without knowing what you're looking for. To nail it:
Make sure you can recreate the error. This helps you figure out the pattern, like Sherlock with his magnifying glass.
Read those pesky error messages. They're not just random words, they're breadcrumbs leading to the bug's hideout.
Jot down the symptoms like you're keeping a diary of your code's drama. When does it misbehave? What does it do? Knowing these will help you track down the troublemaker.
And remember, if the error message is as clear as mud, hit up Google. Someone out there has probably already had a tête-à-tête with the same bug and posted about it online.
2. Embrace the Debugger, Your New Bestie
This one's a game-changer, a tool that lets you peek into your code's soul while it's running. Here's the lowdown on using a debugger like a boss:
Set up some breakpoints, like telling your code, "Halt right here!" where you think the problem might lurk.
Creep through the code like a ninja, one line at a time, with "Step Over" or "Step Into."
Snoop on those variables. Are they playing nice with each other? Are they the values you expect them to be?
For the big guns, use conditional breakpoints. They're like having your code raise a red flag when something fishy happens, like a variable going bananas.
There are some rad tools out there, like Chrome DevTools for JavaScript fans or PDB for Python peeps. And if you're into fancy schmancy stuff, your fave IDEs like Visual Studio Code or IntelliJ IDEA have built-in debuggers that make your life a breeze.
Pro Tip:
Don't be a noob, learn the keyboard shortcuts for your debugger. It'll make you feel like a coding wizard and save you so much time.
So, roll up your sleeves, get cozy with these techniques, and watch those bugs shiver in their boots when you come for them. Happy coding! 💻🐛💥
3. Leverage Logging
So, logging is basically like leaving breadcrumbs in your code, but for debugging. It's super helpful when you've got a pesky bug that just won't show up when you're using a fancy schmancy debugger. By sprinkling some log statements here and there, you can keep an eye on what your program's up to without actually stopping it.
Tips for Logging Like a Pro:
Pick the Right Log Level: There's a bunch of them, like DEBUG, INFO, WARNING, and ERROR, so use 'em wisely to keep your logs organized.
Log What Matters: Focus on the juicy bits, like those pesky if-statements, loops, or when you're chatting with some external API.
Don't Spam Your Logs: Less is more, buddy. Too many logs and you're just gonna get lost in the noise.
Make 'Em Look Nice: Throw in some timestamps, function names, and line numbers. It's like giving your logs a fancy outfit for a night out.
Example:
If you're coding in Python, it's as easy as pie with logging.debug("This is what x is up to: {}".format(x))
. In JavaScript, just console.log("I'm working on user data with ID:", userId)
.
And the best part? You can save these logs for later, like a diary entry, and check 'em out when you're trying to figure out what went wrong in the wild world of production.
4. Break It Down
When you've got a bug that's playing hide and seek in a forest of code, it's time to go minimalist. Break your code into bite-sized chunks to figure out where the gremlin is hiding.
How to Make It Less Overwhelming:
Use Comments: Comment out parts of your code to see if the error goes away. Start with the least amount of code and build back up like you're playing with Lego.
Binary Search: Cut your code in half and test. If the bug's not there, repeat with the other half. It's like finding a needle in a haystack, but you're chopping the haystack in two each time.
Test the Tiny Stuff: Write little test scripts for each piece of your code. It's like giving them a pop quiz to see if they're playing nice.
Example:
If some big ol' function is causing you grief, chop it up into little helper functions and test each one solo.
Remember to keep a safe copy of your code before you go chopping it up. Use something like Git so you don't lose your marbles.
5. Chat with Mr. Duck
Okay, this one might make you feel like you're losing it, but it works. Imagine you've got a rubber duck (or any object that'll listen) who's a newbie coder.
How to Rubber Duck Debug:
Treat the duck like it's new to the code-talking game.
Walk through your code line by line, explaining what each bit is supposed to do.
Tell the duck about the error and why you think it's happening. It's like therapy for your code woes.
You'll be shocked how often just talking it out makes the lightbulb go off above your head.
Pro Tip:
If you don't have a duck (or you're feeling shy), a pal, a voice note, or even just talking to yourself works.
Final Words
Debugging can be a real headache, but it's a skill that gets easier the more you do it. With these five tricks up your sleeve, you'll be zipping through bugs like a pro in no time. Here's a quick cheat sheet:
Get cozy with the problem.
Use a debug tool to take a peek at your code.
Keep tabs on your code with logging.
Simplify your code to spot the troublemaker.
Give the rubber duck a pep talk.
And remember, every bug is just a chance to become a better dev. So, next time you're knee-deep in code issues, don't despair—you're just leveling up! Happy bug hunting!
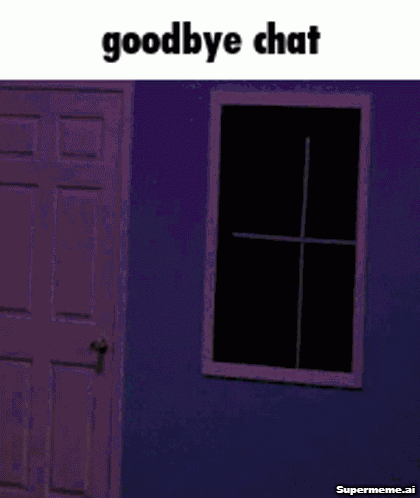